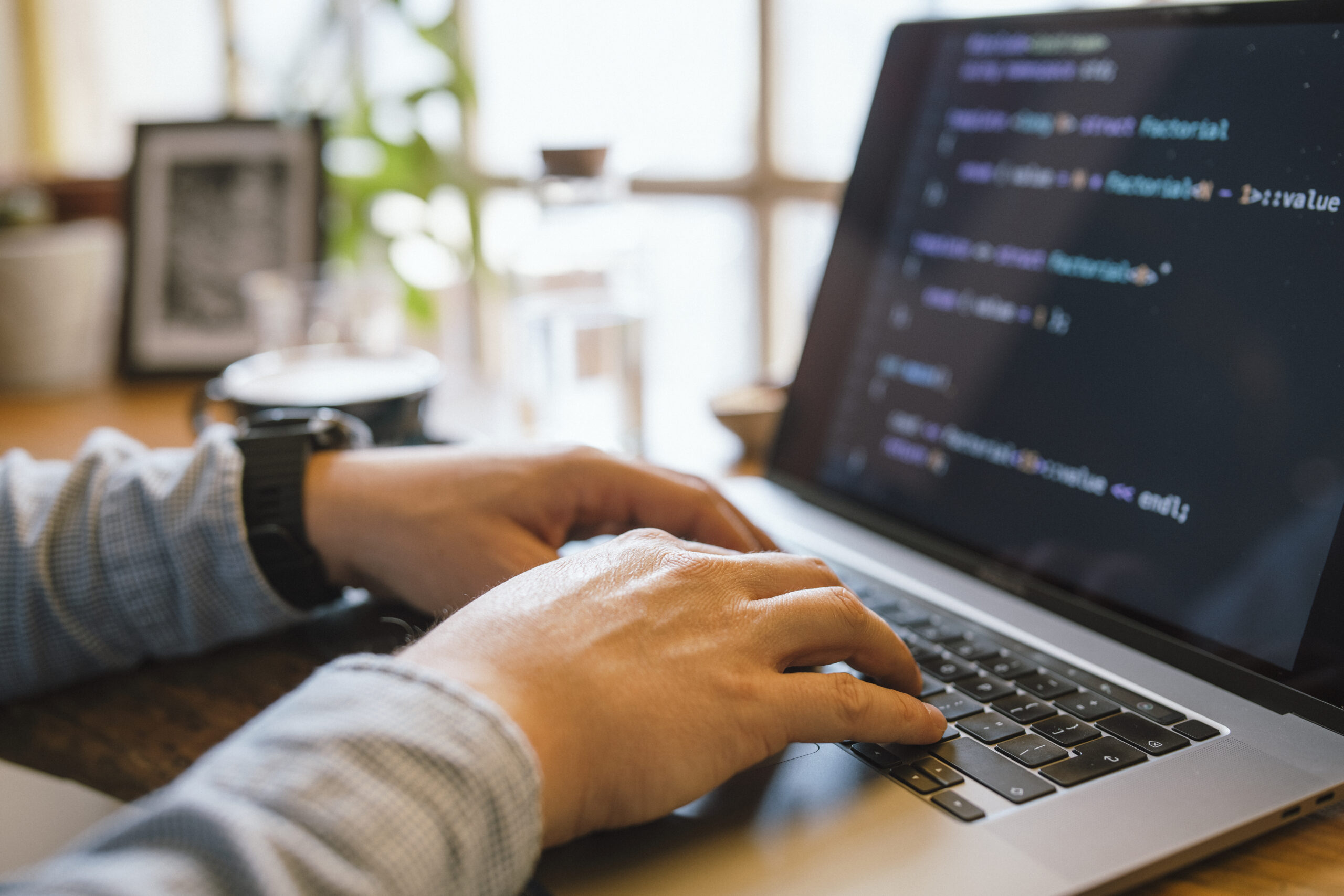
Debugging is One of the more essential — nevertheless generally overlooked — abilities within a developer’s toolkit. It's actually not almost correcting damaged code; it’s about being familiar with how and why matters go wrong, and Studying to Feel methodically to solve difficulties effectively. No matter whether you're a novice or even a seasoned developer, sharpening your debugging expertise can preserve hrs of aggravation and significantly enhance your productivity. Listed here are several strategies to help builders stage up their debugging match by me, Gustavo Woltmann.
Master Your Resources
One of the fastest strategies developers can elevate their debugging abilities is by mastering the tools they use everyday. When producing code is a single A part of development, recognizing tips on how to communicate with it successfully all through execution is Similarly crucial. Modern progress environments arrive equipped with highly effective debugging capabilities — but many builders only scratch the surface of what these applications can do.
Take, for example, an Integrated Development Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications help you set breakpoints, inspect the worth of variables at runtime, stage through code line by line, and in many cases modify code within the fly. When used correctly, they Enable you to observe precisely how your code behaves all through execution, which can be invaluable for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-finish builders. They permit you to inspect the DOM, watch network requests, see authentic-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can flip discouraging UI problems into workable tasks.
For backend or technique-level builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep control in excess of operating processes and memory administration. Studying these instruments may have a steeper Mastering curve but pays off when debugging overall performance issues, memory leaks, or segmentation faults.
Over and above your IDE or debugger, come to be comfortable with Edition Regulate systems like Git to grasp code record, discover the exact second bugs ended up released, and isolate problematic variations.
Ultimately, mastering your resources implies heading over and above default options and shortcuts — it’s about producing an personal familiarity with your progress ecosystem so that when issues arise, you’re not lost at midnight. The better you understand your equipment, the greater time you may expend solving the particular problem rather than fumbling as a result of the procedure.
Reproduce the situation
One of the more important — and sometimes neglected — measures in successful debugging is reproducing the condition. Right before leaping to the code or producing guesses, developers have to have to produce a regular surroundings or scenario where the bug reliably seems. With no reproducibility, fixing a bug becomes a activity of probability, usually leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as feasible. Question concerns like: What steps resulted in the issue? Which natural environment was it in — growth, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it gets to isolate the exact ailments below which the bug takes place.
After you’ve gathered ample info, endeavor to recreate the trouble in your neighborhood atmosphere. This may imply inputting the exact same information, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, take into consideration creating automatic tests that replicate the edge conditions or state transitions included. These checks not just enable expose the issue but in addition reduce regressions Later on.
From time to time, The difficulty could be natural environment-specific — it would materialize only on particular running units, browsers, or under certain configurations. Working with applications like virtual machines, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these bugs.
Reproducing the problem isn’t just a phase — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be now midway to fixing it. With a reproducible situation, You can utilize your debugging equipment far more properly, take a look at probable fixes safely and securely, and converse additional Plainly with the staff or people. It turns an summary grievance into a concrete challenge — and that’s where developers prosper.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when one thing goes Incorrect. Instead of seeing them as frustrating interruptions, builders really should understand to deal with error messages as immediate communications through the program. They usually tell you what precisely took place, in which it happened, and in some cases even why it took place — if you understand how to interpret them.
Begin by examining the concept very carefully and in whole. A lot of developers, specially when underneath time stress, look at the 1st line and right away start building assumptions. But deeper during the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — browse and recognize them first.
Split the error down into areas. Is it a syntax error, a runtime exception, or a logic error? Will it level to a selected file and line amount? What module or functionality induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Understanding to acknowledge these can significantly hasten your debugging process.
Some problems are obscure or generic, As well as in These situations, it’s very important to examine the context through which the mistake occurred. Examine linked log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede much larger challenges and provide hints about prospective bugs.
Eventually, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties a lot quicker, reduce debugging time, and become a much more productive and self-confident developer.
Use Logging Correctly
Logging is Among the most impressive tools in a developer’s debugging toolkit. When utilized successfully, it offers true-time insights into how an software behaves, supporting you fully grasp what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
A superb logging approach begins with being aware of what to log and at here what degree. Frequent logging stages contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for specific diagnostic data for the duration of growth, Information for common events (like successful get started-ups), Alert for prospective problems that don’t break the applying, ERROR for real problems, and Lethal if the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and decelerate your method. Focus on critical activities, state improvements, input/output values, and critical final decision points in the code.
Format your log messages clearly and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Particularly precious in production environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. Which has a nicely-considered-out logging approach, you'll be able to lessen the time it takes to spot difficulties, gain further visibility into your purposes, and improve the All round maintainability and trustworthiness within your code.
Believe Just like a Detective
Debugging is not merely a technological job—it's a kind of investigation. To correctly identify and resolve bugs, developers ought to solution the process like a detective solving a mystery. This frame of mind can help stop working elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the indications of the problem: mistake messages, incorrect output, or performance problems. Much like a detective surveys a crime scene, gather as much related info as you are able to without having jumping to conclusions. Use logs, check instances, and user reports to piece together a transparent photograph of what’s occurring.
Following, kind hypotheses. Request oneself: What could possibly be leading to this behavior? Have any changes a short while ago been built to your codebase? Has this situation transpired prior to under identical situation? The purpose is always to narrow down alternatives and establish likely culprits.
Then, check your theories systematically. Try to recreate the condition in the controlled ecosystem. When you suspect a particular function or part, isolate it and verify if the issue persists. Similar to a detective conducting interviews, talk to your code issues and Permit the outcomes guide you nearer to the truth.
Fork out close notice to smaller specifics. Bugs often cover within the the very least expected locations—similar to a missing semicolon, an off-by-a person error, or simply a race issue. Be thorough and individual, resisting the urge to patch the issue with no fully comprehension it. Temporary fixes may possibly hide the true trouble, only for it to resurface afterwards.
Lastly, retain notes on what you experimented with and learned. Just as detectives log their investigations, documenting your debugging system can conserve time for long run issues and support Many others realize your reasoning.
By imagining like a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more practical at uncovering concealed problems in sophisticated devices.
Write Tests
Composing assessments is among the simplest ways to enhance your debugging capabilities and Over-all enhancement efficiency. Tests not just aid catch bugs early and also function a security Internet that provides you assurance when earning changes for your codebase. A effectively-examined application is easier to debug since it permits you to pinpoint just the place and when a difficulty happens.
Begin with unit exams, which concentrate on personal functions or modules. These little, isolated tests can quickly expose whether a selected bit of logic is Performing as predicted. Each time a examination fails, you right away know exactly where to appear, significantly reducing some time expended debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear soon after Formerly becoming fixed.
Future, integrate integration tests and close-to-conclusion exams into your workflow. These assist ensure that several areas of your application get the job done collectively smoothly. They’re significantly valuable for catching bugs that arise in sophisticated systems with many components or products and services interacting. If anything breaks, your tests can inform you which Portion of the pipeline unsuccessful and below what disorders.
Composing tests also forces you to definitely Believe critically regarding your code. To test a attribute correctly, you require to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. Once the take a look at fails consistently, you'll be able to deal with fixing the bug and look at your exam pass when the issue is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, creating assessments turns debugging from the frustrating guessing recreation into a structured and predictable course of action—helping you catch a lot more bugs, speedier plus more reliably.
Take Breaks
When debugging a tricky concern, it’s uncomplicated to be immersed in the condition—staring at your screen for hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging tools is simply stepping away. Taking breaks assists you reset your thoughts, minimize disappointment, and sometimes see The problem from a new viewpoint.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain turns into significantly less effective at problem-resolving. A brief stroll, a coffee break, or simply switching to a unique process for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially through more time debugging sessions. Sitting down in front of a screen, mentally caught, is not just unproductive but also draining. Stepping absent enables you to return with renewed energy and also a clearer attitude. You might quickly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, extend, or do something unrelated to code. It could feel counterintuitive, Specially under restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks just isn't an indication of weakness—it’s a wise tactic. It gives your brain Place to breathe, increases your viewpoint, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is a component of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—it's an opportunity to increase for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you some thing useful in case you go to the trouble to replicate and analyze what went Incorrect.
Commence by asking by yourself some vital thoughts once the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding habits moving ahead.
Documenting bugs will also be a wonderful practice. Retain a developer journal or retain a log where you Be aware down bugs you’ve encountered, how you solved them, and what you acquired. Eventually, you’ll begin to see designs—recurring problems or common mistakes—you could proactively prevent.
In crew environments, sharing Everything you've discovered from the bug with the peers can be Primarily highly effective. No matter whether it’s through a Slack information, a short write-up, or A fast information-sharing session, assisting Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as important aspects of your advancement journey. After all, many of the very best builders aren't those who write best code, but those who continually learn from their problems.
Eventually, Each and every bug you take care of adds a different layer to your ability established. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities takes time, apply, and endurance — though the payoff is huge. It can make you a more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.